In this c program:
- The user is prompted to enter the temperature in Fahrenheit.
- The program calculates the equivalent temperature in Celsius using the formula
(Fahrenheit - 32) * 5 / 9
. - The result is then printed, showing the temperature in Celsius.
You can run this program, enter a temperature in Fahrenheit, and it will display the equivalent temperature in Celsius.
Fahrenheit to Celsius :
#include<stdio.h>
#include<conio.h>
int main()
{
float f,c;
printf(“Enter The Fahrenheit Value : “);
scanf(“%f”,&f);
c=(f-32)*5/9;
printf(“Celsius Value : %0.3f”,c);
}
Output :
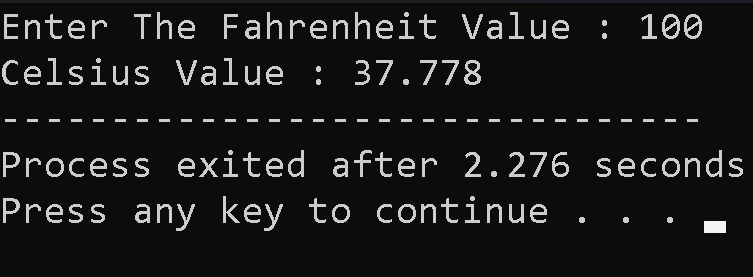
To convert Celsius to Fahrenheit in a C program, you can use the formula:
F=9/5×C + 32
Here’s an example C program that takes a temperature in Celsius as input and converts it to Fahrenheit
Celsius to Fahrenheit :
#include<stdio.h>
#include<conio.h>
int main()
{
float f,c;
printf(“Enter The Celsius Value : “);
scanf(“%f”,&c);
f=(c*9/5)+32;
printf(“Fahrenheit Value : %0.3f”,f);
}
Output :
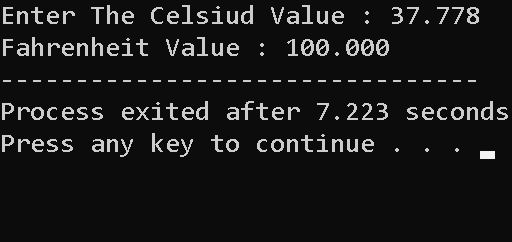