Below is a simple example of a C program for employee salary calculation. This program takes basic salary as input and calculates the gross salary by adding allowances and deducting taxes.
In this program:
- The user is prompted to enter the basic salary.
- The program calculates the house rent allowance (HRA), dearness allowance (DA), and tax based on certain percentages of the basic salary.
- The gross salary is then calculated by adding HRA and DA and subtracting the tax.
- Finally, the program prints the basic salary, HRA, DA, tax, and the gross salary.
Note: This is a simple example, and in a real-world scenario, you might need to consider more factors such as other allowances, deductions, and applicable tax rules.
Program :
#include<stdio.h>
#include<conio.h>
int main()
{
float a,da,hra,ta,ma,gross,pf,sal;
printf(“Enter Basic Pay : “);
scanf(“%f”,&a);
da=(a*18)/100;
printf(“\nDearness Allowance(DA) is : %0.2f”,da);
ta=(a*15)/100;
printf(“\nTravel Allowance(TA) is : %0.2f”,ta);
hra=(a*10)/100;
printf(“\nHouse Rent Allowance(HRA) is : %0.2f”,hra);
ma=(a*15)/100;
printf(“\nMedical Allowance(MA) is : %0.2f”,ma);
gross=a+da+ta+hra+ma;
printf(“\nGross salary : %0.2f”,gross);
pf=(gross*13)/100;
printf(“\nProvident Fund(Pf) is : %0.2f”,pf);
sal=gross-pf;
printf(“\nNet Salary is : %0.2f”,sal);
}
Output :
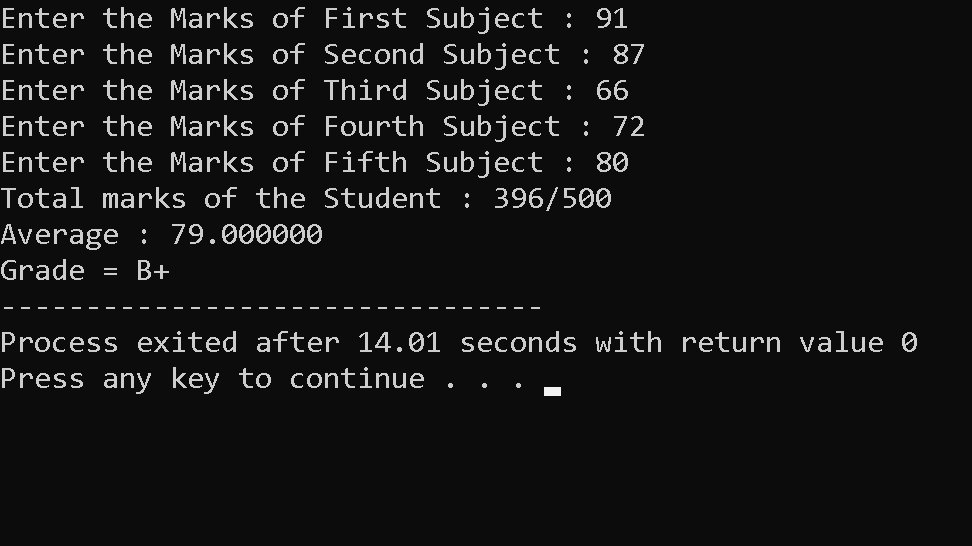
sneeriest xyandanxvurulmus.xZR8LyKk2ZVQ