This c program allows the user to choose between a rectangle, circle, and triangle. It then prompts the user for the required parameters (length and width for a rectangle, radius for a circle, and base and height for a triangle) and calculates and prints the area based on the chosen shape. The ‘switch
‘ statement is used to handle the different cases for each shape.
Program :
#include <stdio.h>
#include <conio.h>
#include <math.h>
int main ()
{
int choice;
float a,r,t1,t2,s,d1,d2,p,h;
printf(“Enter 1 for area of circle\n”);
printf(“Enter 2 for area of Triangle\n”);
printf(“Enter 3 for area of Square\n”);
printf(“Enter 4 for area of Rhombus\n”);
printf(“Enter 5 for area of Pentagon\n”);
printf(“Enter 6 for area of Hexagon\n”);
printf(“Enter your choice : “);
scanf(“%d”,&choice);
switch(choice)
{
case 1:
printf(“Enter the value of radius : “);
scanf(“%f”,&r);
//Area=3.14*Radius*Radius
a=3.14*r*r;
printf(“\nArea of the Circle : %0.2f”,a);
break;
case 2:
printf(“Enter the base of Triangle : “);
scanf(“%f”,&t1);
printf(“Enter the height of Triangle : “);
scanf(“%f”,&t2);
//Area=(base*height)/2
a=(t1*t2)/2;
printf(“\nArea of the Triangle: %0.2f”,a);
break;
case 3:
printf(“Enter the Length of Side : “);
scanf(“%f”,&s);
//Area=(Side*Side)
a=(s*s);
printf(“\nArea of the Square : %0.2f”,a);
break;
case 4:
printf(“Enter the Length of first Diagonal : “);
scanf(“%f”,&d1);
printf(“Enter the Length of second Diagonal : “);
scanf(“%f”,&d2);
//Area=(Diagonal1*Diagonal2)/2
a=(d1*d2)/2;
printf(“\nArea of the Rhombus: %0.2f”,a);
break;
case 5:
printf(“Enter the Length of Side : “);
scanf(“%f”,&p);
a=(sqrt(5*(5+2*sqrt(5)))*p*p)/4;
printf(“\nArea of the Pentagon: %0.2f”,a);
break;
case 6:
printf(“Enter the Length of Side : “);
scanf(“%f”,&h);
a=(3*sqrt(3)*h*h)/2;
printf(“\nArea of the Hexagon: %0.2f”,a);
break;
}
return 0;
}
Output :
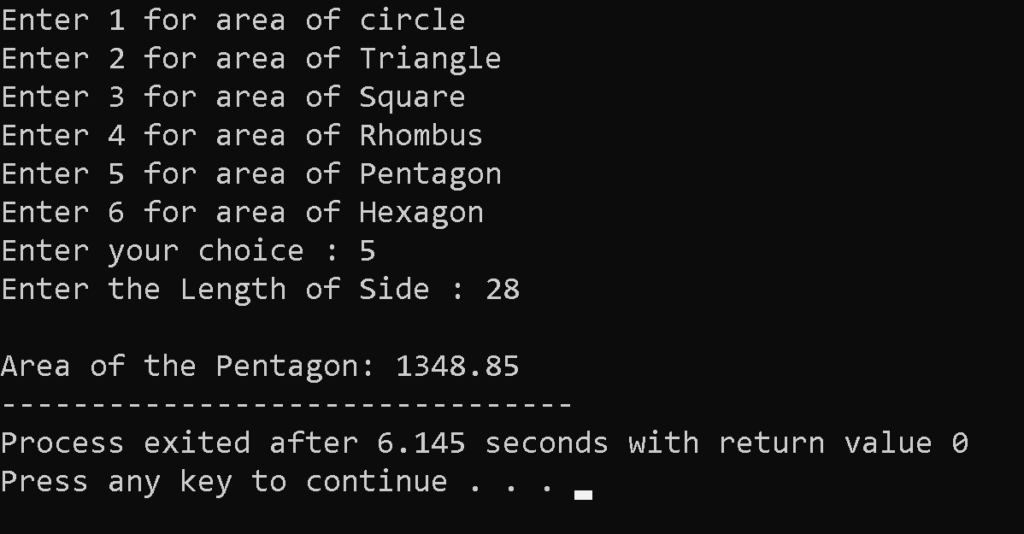
vurcazkircazpatliycaz.JGoXaBLT93yt
bundies xyandanxvurulmus.Bxn3nTFzGdaC