Sure, I can provide you with a simple implementation of the Shell Sort algorithm in C. Shell Sort is an in-place comparison-based sorting algorithm that sorts elements far apart from each other and then progressively reduces the gap between elements to be compared. Here’s a basic implementation in C Program :
Program :
#include<stdio.h>
#include<conio.h>
void shellsort(int a[],int n)
{
int j,i,m,mid;
for(m=n/2;m>0;m/=2)
{
for(j=m;j<n;j++)
{
for(i=j-m;i>=0;i-=m)
{
if(a[i+m]>=a[i])break;
else
{
mid=a[i];
a[i]=a[i+m];
a[i+m]=mid;
}
}
}
}
}
void main()
{
int a[10],i,n;
printf(“Enter the number of Elements : “);
scanf(“%d”,&n);
for(i=0;i<n;i++)
{
printf(“Element%d : “,i+1);
scanf(“%d”,&a[i]);
}
printf(“\nArray before sorting :”);
for(i=0;i<n;i++)
{
printf(“\t%d”,a[i]);
}
shellsort(a,n);
printf(“\nArray after sorting : “);
for(i=0;i<n;i++)
{
printf(“\t%d”,a[i]);
}
getch();
}
Output :
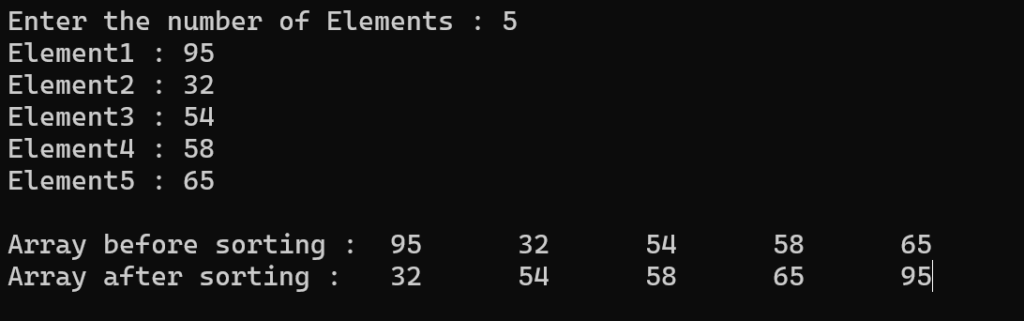