Below is a C program that prints various alphabet patterns. Each pattern corresponds to a different letter of the alphabet.
Program 1 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=’A’;x<=n;x++)
{
printf(“\n%c”,x);
}
return 0;
}
Output :
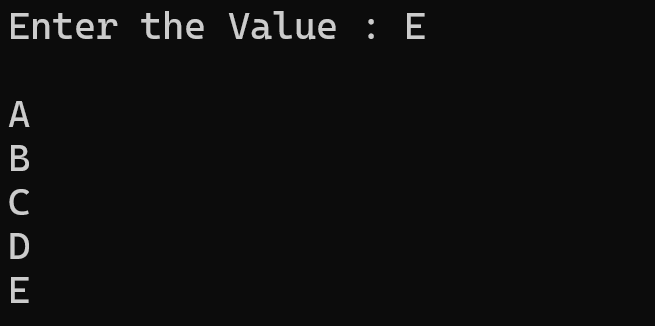
Program 2 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=’A’;x<=n;x++)
{
printf(“%c “,x);
}
return 0;
}
Output :
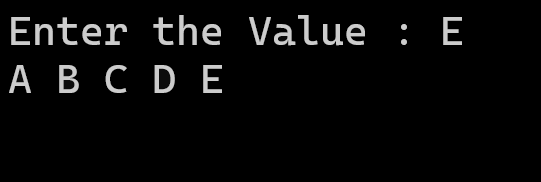
Program 3 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=’A’;x<=n;x++)
{
for(y=’A’;y<=n;y++)
{
printf(” %c”,x);
}
printf(“\n”);
}
}
Output :

Program 4 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,z,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=n;x>=’A’;x–)
{
for(y=n-1;y>=x;y–)
{
printf(” “);
}
for(z=’A’;z<=x;z++)
{
printf(“%c “,x);
}
printf(“\n”);
}
}
Output :
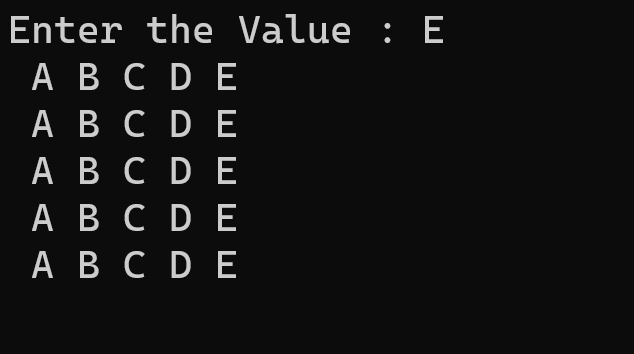
Program 5 :
#include<stdio.h>
#include<stdio.h>
int main()
{
char x,y,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=’A’;x<=n;x++)
{
for(y=’A’;y<=x;y++)
{
printf(” %c”,y);
}
printf(“\n”);
}
}
Output :
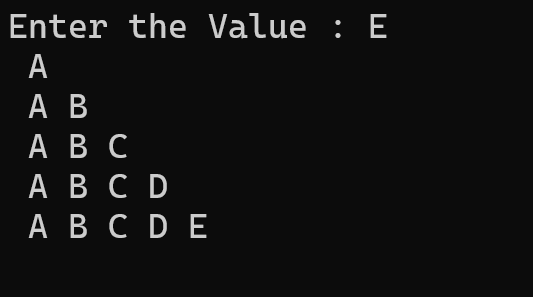
Program 6 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,z,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=n;x>=’A’;x–)
{
for(y=n-1;y>=x;y–)
{
printf(” “);
}
for(z=’A’;z<=x;z++)
{
printf(“%c “,x);
}
printf(“\n”);
}
}
Output :
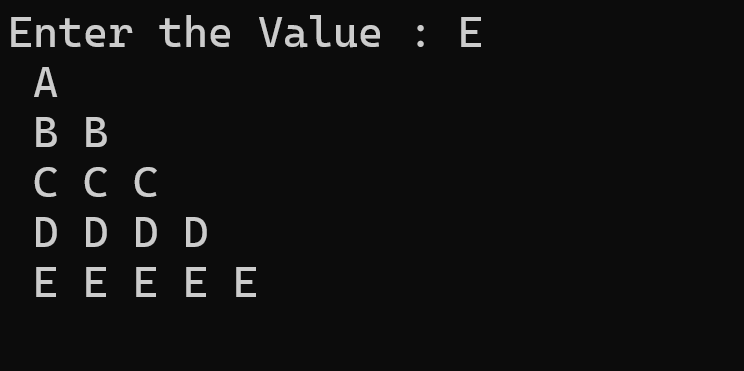
Program 7 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=’A’;x<=n;x++)
{
for(y=n;y>=x;y–)
{
printf(“%c “,y);
}
printf(“\n”);
}
}
Output :

Program 8 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=’A’;x<=n;x++)
{
for(y=n;y>=x;y–)
{
printf(“%c “,x);
}
printf(“\n”);
}
}
Output :
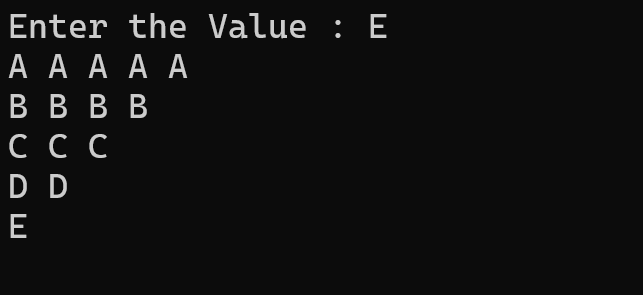
Program 9 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,n,z;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=’A’;x<=n;x++)
{
for(y=n-1;y>=x;y–)
{
printf(” “);
}
for(z=’A’;z<=x;z++)
{
printf(“%c”,x);
}
printf(“\n”);
}
}
Output :
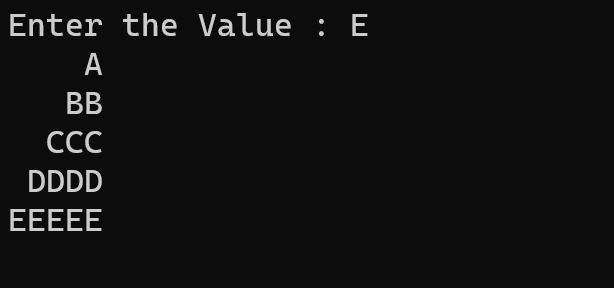
Program 10 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,n,z;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=’A’;x<=n;x++)
{
for(y=n-1;y>=x;y–)
{
printf(” “);
}
for(z=’A’;z<=x;z++)
{
printf(“%c”,z);
}
printf(“\n”);
}
}
Output :
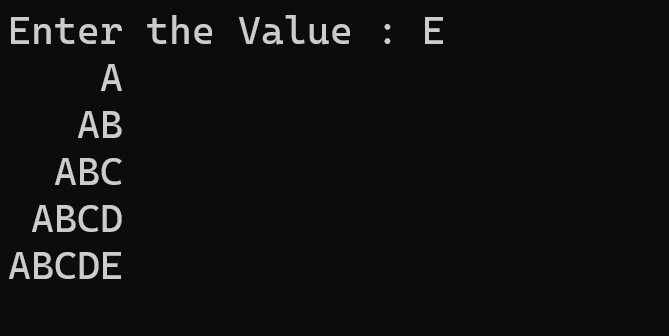
Program 11 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,z,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=n;x>=’A’;x–)
{
for(y=n-1;y>=x;y–)
{
printf(” “);
}
for(z=’A’;z<=x;z++)
{
printf(“%c”,x);
}
printf(“\n”);
}
}
Output :
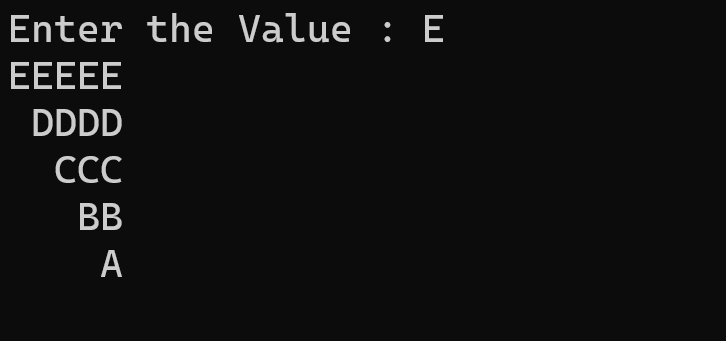
Program 12 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,z,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=n;x>=’A’;x–)
{
for(y=n-1;y>=x;y–)
{
printf(” “);
}
for(z=’A’;z<=x;z++)
{
printf(“%c”,z);
}
printf(“\n”);
}
}
Output :
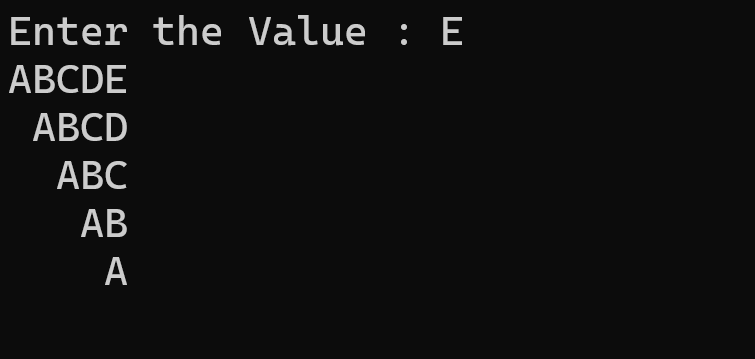
Program 13 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=n;x>=’A’;x–)
{
for(y=’A’;y<=x;y++)
{
printf(“%c”,y);
}
printf(“\n”);
}
}
Output :

Program 14 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=n;x>=’A’;x–)
{
for(y=’A’;y<=x;y++)
{
printf(“%c”,x);
}
printf(“\n”);
}
}
Output :

Program 15 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,z,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=n;x>=’A’;x–)
{
for(y=n-1;y>=x;y–)
{
printf(” “);
}
for(z=’A’;z<=x;z++)
{
printf(“%c “,x);
}
printf(“\n”);
}
}
Output :
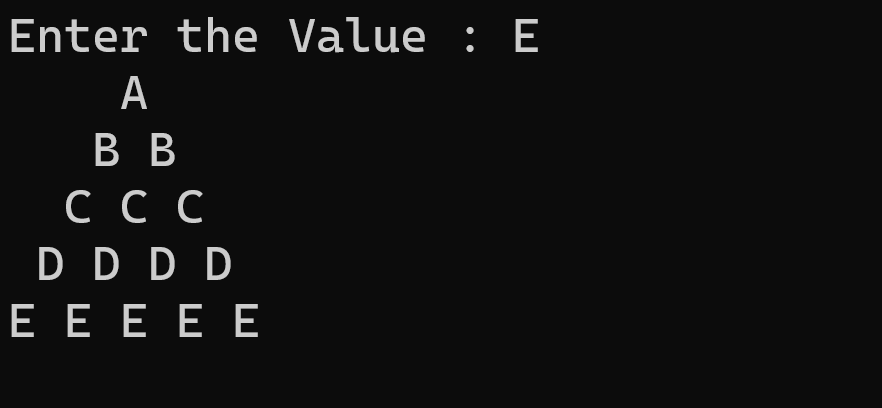
Program 16 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,z,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=n;x>=’A’;x–)
{
for(y=n-1;y>=x;y–)
{
printf(” “);
}
for(z=’A’;z<=x;z++)
{
printf(“%c “,x);
}
printf(“\n”);
}
}
Output :
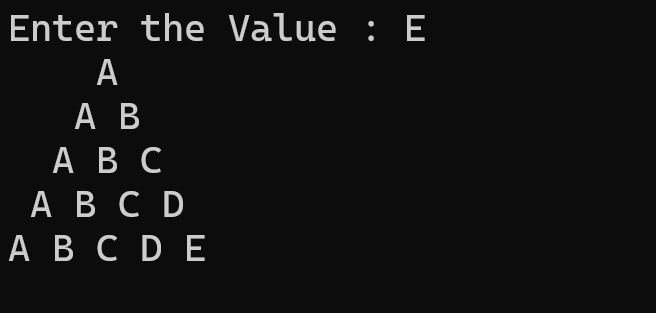
Program 17 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,z,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=n;x>=’A’;x–)
{
for(y=n-1;y>=x;y–)
{
printf(” “);
}
for(z=’A’;z<=x;z++)
{
printf(“%c “,x);
}
printf(“\n”);
}
}
Output :
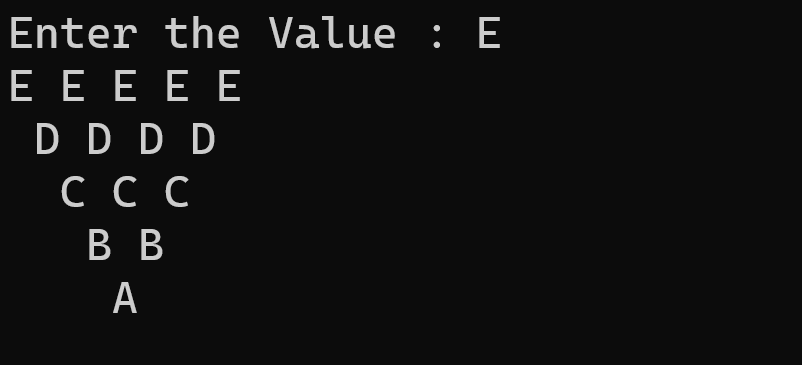
Program 18 :
#include<stdio.h>
#include<conio.h>
int main()
{
char x,y,z,n;
printf(“Enter the Value : “);
scanf(“%c”,&n);
for(x=n;x>=’A’;x–)
{
for(y=n-1;y>=x;y–)
{
printf(” “);
}
for(z=’A’;z<=x;z++)
{
printf(“%c “,z);
}
printf(“\n”);
}
}
Output :
